It might be required to initialize the state of the Qubit before performing operations on it. In this chapter of the Qiskit Tutorial, you will learn about how you can initialize the state of a Qubit.
By default, the state of a Qubit is |0>
. You can initialize a Qubit into whatever state you want.
Initializing the State to |1>
Initializing the Qubit into state |1>
is quite straight forward. You can make use of X Gate on the state that is initialized in the state |0>
.
This works because the X Gate exchanges the coefficients of |0>
and |1>
in the equation defining the state of the Qubit. A Qubit in the state |0>
can be defined as ψ = 1 |0> + 0 |1>
. After applying the X Gate, the state of the Qubit changes to ψ = 1 |0> + 0 |1> = |1>
Initializing into any State
You may not always want to initialize a Qubit into either |0>
or |1>
state. You can initialize a Qubit into whatever state you want. This can be achieved by calling the initialize()
method on the Quantum Circuit. The amplitudes(coefficients of |0>
and |1>
) are passed to the initialize()
method as a list along with the index of the Qubit which needs to be initialized with this state.
Note– The amplitudes of |0>
and |1>
, commonly referred to as α and β are complex numbers. Therefore, the list representing the state vector can contain complex numbers.
Example
In this example, we will create a 2 Qubit Quantum Circuit and initialize the state of first Qubit(at index 0) into a state of superposition. The Qubit will be initialized into the state ψ = 1/sqrt(2) |0> + 1/sqrt(2) |1>
.
To initialize the Qubit with the given state, the state vector [1/sqrt(2), 1/sqrt(2)]
is passed to the initialize()
method called on the Quantum Circuit along with the index of the Qubit which needs to be initialized.
import numpy as np
from qiskit import QuantumCircuit
qc = QuantumCircuit(2)
# Initializing the 1st Qubit with the given vector
qc.initialize([1/np.sqrt(2), 1/np.sqrt(2)], 0)
# Drawing the Quantum Circuit
qc.draw()
The output of this will be-
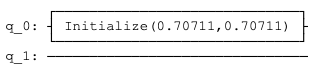
Note– Since the sum of squares of α and β is 1, the sum of the squares of both the elements of the vector also need to 1. Else, the following error is raised-
QiskitError: 'Sum of amplitudes-squared does not equal one.'
You can also initialize multiple Qubits into a state of superposition. Learn More