Unitary Simulator is a simulator provided by Qiskit that calculates and returns the unitary matrix that represents the Quantum Circuit. It is useful when the unitary matrix corresponding to the Quantum Circuit is to be known. It cannot be used to visualize the state of the Qubits or to run the Quantum Circuit and perform measurements. In this chapter of the Qiskit tutorial, you will learn about Unitary Simulator and how you can use the Unitary Simulator from the BasicAer
module of qiskit.
Since Unitary Simulator calculates and returns the unitary matrix corresponding to the Quantum Circuit, the Quantum Circuit which is to be simulated on the Unitary Simulator should not contain any non-unitary instruction. Non-unitary instructions include reset instruction, initialization instructions, measurement instructions, and classical conditional operations.
Selecting the Unitary Simulator
The Unitary Simulator from BasicAer
module can be selected using the get_backend()
function.
from qiskit import BasicAer
backend = BasicAer.get_backend('unitary_simulator')
Creating a Quantum Circuit
Unitary simulator is useful only when it is important to calculate the unitary matrix corresponding to the Quantum Circuit.
from qiskit import QuantumCircuit
qc = QuantumCircuit(2)
qc.h(0)
qc.h(1)
Note– No measurement instruction is a part of the Quantum Circuit. Since the only job of bits is to store the results of Qubits once they are measured, no bits are required in the Quantum Circuit.
The Quantum Circuit specified above puts both the Qubits in the state of superposition. The state ψ
of the Quantum Circuit can be written as-
ψ = 1/sqrt(4) [ |00> + |01> + |10> + |11> ]
Note– sqrt(4)
denotes square-root of 4, i.e, 2.
Simulating Quantum Circuit through Unitary Simulator
We will stimulate the Quantum Circuit we just created.
Creating a Job
First we execute the Quantum Circuit we created on the Unitary Simulator backend. This creates a job to execute the Quantum Circuit which is stored as an object in the variable job
.
from qiskit.execute_function import execute
job = execute(qc, backend)
Getting Results
job
object contains information related to the job that was executed on the Unitary Simulator. The result of the simulation can be obtained by calling the result()
method on job
. The result is stored as an object in the variable result
.
result = job.result()
The state of Qubits can be obtained from the result
variable by calling the get_unitary()
methods on result
.
unitary_mat = result.get_unitary()
# Print the unitary matrix
print(unitary_mat)
The output of this will be-
array([[ 0.5+0.0000000e+00j, 0.5-6.1232340e-17j, 0.5-6.1232340e-17j, 0.5-1.2246468e-16j], [ 0.5+0.0000000e+00j, -0.5+6.1232340e-17j, 0.5-6.1232340e-17j, -0.5+1.2246468e-16j], [ 0.5+0.0000000e+00j, 0.5-6.1232340e-17j, -0.5+6.1232340e-17j, -0.5+1.2246468e-16j], [ 0.5+0.0000000e+00j, -0.5+6.1232340e-17j, -0.5+6.1232340e-17j, 0.5-1.2246468e-16j]])
This is the same as the expected unitary matrix of the circuit-
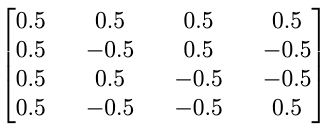
Note– If the Quantum Circuit that is simulated on the Unitary Simulator contains any non-unitary operation such as measurement, initialization, reset, or classical conditional operation, the following error will be raised-
BasicAerError: 'Unsupported "%s" instruction "%s" in circuit "%s" unitary_simulator reset circuit-267'