In this chapter, you will learn how to crop and paste sections of an image using the Pillow library.
crop()
The crop()
method is used to crop a region from an image. It returns the cropped image which is an object of the Image
class.
Syntax:
image.crop((left, upper, right, lower))
The figure below shows how the coordinates of the region to be cropped are passed to crop()
. In the figure below, grey represents the image and black represents the region being cropped from the image.
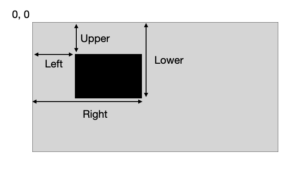
The region which has to be cropped from the original image is passed to crop()
as a tuple. The tuple contains 4 elements- left, upper, right, and lower. These 4 elements specify the size and location of the cropped image. The left and upper specify the x and y coordinates of the top-left corner while the right and lower specify the x and y coordinates of the bottom-right corner of the region we are cropping. The top-left corner of the image has coordinates 0,0.
Note– The illustration above shows how to crop a region from an image. However, both crop and paste operations using Pillow are performed in a similar fashion as you will learn soon.
Example
# This is the region to be cropped from the image
region = (800, 800, 1600, 1600)
# cropping a region from the image and storing it as cropped_img
cropped_img = img.crop(region)
cropped_img.show()
The output of this will be-
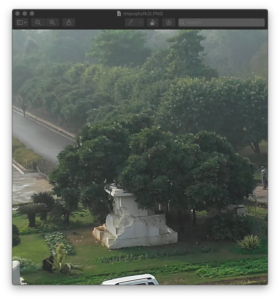
Example
If the elements passed to the crop() are such that, right is less than left or lower is less than upper. Then the resulting image will contain nothing. However, it will still continue to be an object of the Image
class. Furthermore, it cannot be seen using the show()
method or saved using the save()
method.
region_2 = (400, 400, 200, 200)
cropped_img_2 = img.crop(region_2)
print(cropped_img_2.size)
# Outputs- (0, 0)
cropped_img_2.show()
# Outputs- SystemError: tile cannot extend outside image
cropped_img_2.save('noimage.png')
# Outputs- SystemError: tile cannot extend outside image
paste()
The paste()
method is used to paste an image over another image. It does not return the edited image, the image is edited in place.
Syntax:
image.paste(image_to_be_pasted, (left, upper, right, lower))
The new image which is to be pasted on the image is passed to paste()
along with the coordinates of the region(as a tuple). The region where the image is to be pasted is passed as a tuple contains 4 elements- left, upper, right, and lower. These elements specify the region in the same way as they do in crop()
.
Example
In this example, we will paste the region of the image we cropped in the previous example to the image itself.
# This is the region where the cropped image is to be pasted
region_3 = (200, 200, 1000, 1000)
# pasting the cropped region to the image itself
img.paste(cropped_img, region_3)
img.show()
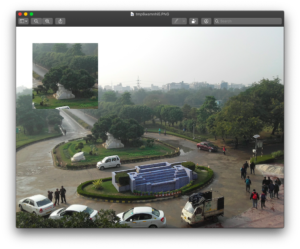
Note– The size of the image which has to be pasted should match exactly with the dimension of the region which is passed to paste()
. Otherwise, a ValueError
is returned.
Instead of passing all the 4 coordinates to paste(), we can just the left and upper coordinates. The right and lower coordinates are calculated by using the size of the image.
Syntax:
image.paste(image_to_be_pasted, (left, upper))
Example
In this example, we will paste the region of the image we cropped in the previous example to the image itself.
# This is the region where the cropped image is to be pasted
region_4 = (200, 200)
# pasting the cropped region to the image itself
img.paste(cropped_img, region_4)
img.show()
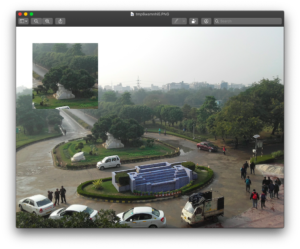