Once operations are performed on Qubits, it is important to measure their values. However, as you are already discussed priviously that measuring a Qubit destroys the state of superposition and the state of the Qubit collapses into either 0 or 1(or more specifically |0>
or |1>
). Therefore, the Qubits can be measured and their values can be stored into classical bits. In this chapter of the Qiskit Tutorial, you will learn about measuring a Qubit in the Quantum Circuit and storing the result in a classical bit.
In this chapter, we will be performing measurements on the following Quantum Circuit-
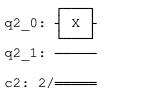
qr=qiskit.QuantumRegister(2)
cr=qiskit.ClassicalRegister(2)
# Creating a Quantum Circuit from qr and cr
circuit=QuantumCircuit(qr, cr)
The first Qubit, q2_0
has a X Gate operating on it, while the second Qubit q2_1
doesn’t have any gate operating on it. You will learn about X Gate in later chapters. Having an understanding of the various gates is not required in this chapter.
Measuring Single Qubit
To measure the state of a single Qubit onto a bit, you can call the measure()
method on the Quantum Circuit and pass it the index of Qubit(which is 0 based) to be measured and the index of classical bit(which is also 0 based) on which the result is to be stored.
Example
In this example, we will measure the value of the 1st Qubit and store the result in the 1st bit.
# Measuring the 1st Qubit(at 0 index) on the 1st bit(at 0 index)
circuit.measure(0, 0)
# Vizualizing the Circuit after measurement
circuit.draw()
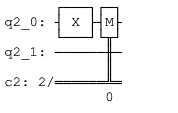
Measuring Multiple Qubits
Instead of measuring one Qubit at once, it is possible to measure multiple Qubits at once. To measure multiple Qubits at once, you can call the measure()
method on the Quantum Circuit and pass it the list of indexes of Qubits you want to measure and the list of indexes of bits you want to measure.
Example
In this example, we will measure the 1st Qubit in the 1st bit and the 2nd Qubit in the 2nd bit.
# Measuring the 1st Qubit in the 1st bit and 2nd Qubit in the 2nd bit
circuit.measure([0, 1], [0, 1])
# Vizualizing the Circuit after measurement
circuit.draw()
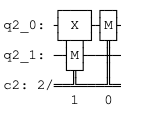
Measuring all Qubits
It is also possible to measure all the Qubits in the Quantum Circuit at once. This can be done by calling the measure_all()
method on the Quantum Circuit.
Also note, that when using measure_all()
method, you don’t need to have classical bits in your Quantum Circuit. measure_all()
method creates classical bits and write measurements onto them.
Example
In this example, we will create a Quantum Circuit with only Qubits and measure all the Qubits of the Quantum Circuit by using the measure_all()
method.
# Creating a Quantum Circuit with 2 Qubits
circuit = QuantumCircuit(2)
# Adding X Gate on Qubit 0
circuit.x(0)
# Measuring all the Qubits at once by using measure_all method
circuit.measure_all()
# Vizualizing the Circuit after measurement
circuit.draw()
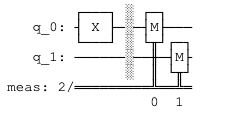
Notice that Qiskit automatically also adds a barrier before measuring the Qubits. You will learn more about this barrier in later chapters.
Note– While it is not necessary to store the measurement of a qubit at a particular index at the corresponding index classical bit, but it is a standard practice to do so. For example, the measurement of Qubit at index 0 is stored in classical bit with index 0, and so on.