Histogram is a preferred, intuitive and easy way to understand and visualize the results of a Quantum Circuit. Qiskit provides inbuilt function to create histogram visualizations. In this chapter of the Qiskit Tutorial, you will learn about how to create histogram visualization by making use of the visualization module of qiskit.
A histogram plots the frequencies of measurements of Qubits. This makes histogram an excellent choice for visualizing the results from QASM Simulator.
Importing Histogram Visualization Function
The plot_histogram()
function of qiskit.visualization
creates Histogram visualization.
from qiskit.visualization import plot_histogram()
Plotting Histogram
The plot_histogram()
method accepts data for plotting histogram in the form of a Python dictionary. The dictionary has the various measurement values as keys and their corresponding frequencies as values.
Example
In the following example, we create a histogram with the following measurement frequencies-
State | Frequency |
---|---|
00 | 0 |
01 | 0 |
10 | 0 |
11 | 1000 |
plot_histogram({'00':0, '01': 0, '10': 0, '11': 1000})
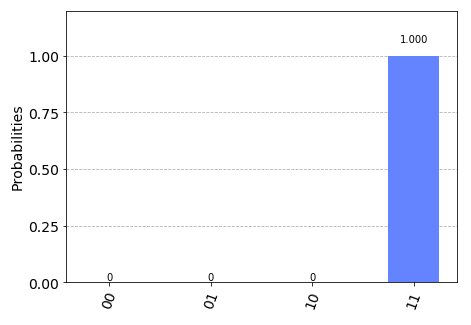
Visualizing QASM Simulator Results on Histogram
In this section, we visualize the results from the QASM Simulator using a histogram.
# Creating a Quantum Circuit to run on the QASM Simulator
qc=QuantumCircuit(2, 2)
# Applying H Gate to both Qubits
qc.h([0,1])
# Measuring Qubits onto respective bits
qc.measure([0, 1], [0, 1])
# Setting Backend to QASM Simulator
backend = BasicAer.get_backend('qasm_simulator')
# Executing the Quantum Circuit
job=execute(qc, backend, shots=10000)
#Get results from job
result = job.result()
# Getting the count of various measurement states
counts = result.get_counts()
# Plotting histogram
plot_histogram(counts)
The output of this will be-
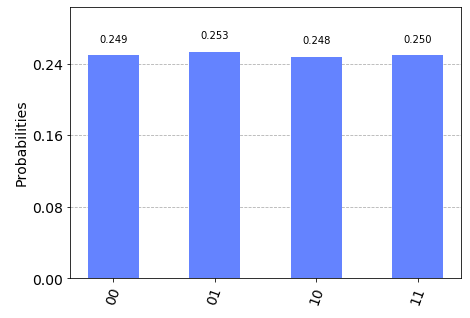
Note– The get_counts()
method returns the counts from the QASM Simulator in the form of a Python dictionary. Therefore, counts
variable can be passed directly to the plot_histogram()
function.
Plotting Multiple Results
It is even possible to plot multiple results on a single histogram by passing a list of Python dictionaries in place of a single dictionary to the plot_histogram()
function.
Example
In the following example, we plot multiple-results on a single histogram.
State | Frequency |
---|---|
00 | 0 |
01 | 0 |
10 | 0 |
11 | 1000 |
State | Frequency |
---|---|
00 | 250 |
01 | 250 |
10 | 250 |
11 | 250 |
# Plotting multiple results in a single histogram
qiskit.visualization.plot_histogram([
{'00':0, '01': 0, '10': 0, '11': 1000},
{'00':250, '01': 250, '10': 250, '11': 250}],
color=['blue', ['red']])
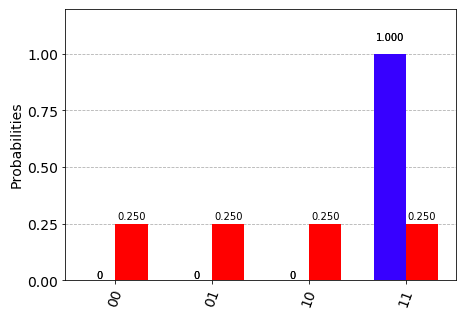
Parameters
The following are the parameters of the plot_histogram()
function-
Parameter | Brief Description |
---|---|
data | It is a dictionary or a list of dictionaries(for multiple results) which contains the data on the frequencies of measurement. It is usually passed as a positional parameter. |
title | It is a string that is used for the plot title. |
legend | A list of strings(one corresponding to each result) to be used for each label of the data. |
bar_labels | A boolean value weather the bars in histogram are to be labeled with probability values . True by default. |
color | A string or a list of strings(for multiple results) that contains the color of the histogram bars. |
figsize | A Tuple that contains the size of the figure in inches. |