The Phase Gate is an important gate in Quantum Computing. Qiskit provides a method for applying Phase Gate on a Qubit. In this chapter of the Qiskit Tutorial, you will learn about Phase Gate and how to apply Phase Gate on a Qubit in Qiskit.
Phase Gate
The Phase Gate acts on a single Qubit. The Phase gate changes the phase of the Qubit by a specified amount. However, in terms of its effect on a state represented in terms of the standard basis pairs |0>
and |1>
, it has no effect on the coefficients of |0>
to |1>
. Hence, the application of Phase Gate does not alter the probabilities of the state of the Qubit collapsing into |0>
or |1>
upon measurement.
The Phase Gate is also known as P Gate.
Note– The Z Gate is a special case of Phase Gate where the parameter(the phase change) is 180° or π radians.
Phase Gate: Bloch Sphere
The P Gate performs a rotation about the Z-axis on the Bloch Sphere by a specified amount(in radians) in the counter-clockwise direction.
Example
In this example, we will look at how the application of Phase gate on the Qubit affects its representation on the Bloch Sphere. Remember how Phase Gate performs a rotation by the specified amount in radians about the Z-axis on the Bloch Sphere.
In the below figure, the Phase Gate is applied on a Qubit in the state Ψ = |+>
with the parameter π/2
, and the resulting state of the Qubit is Ψ = |i>
.
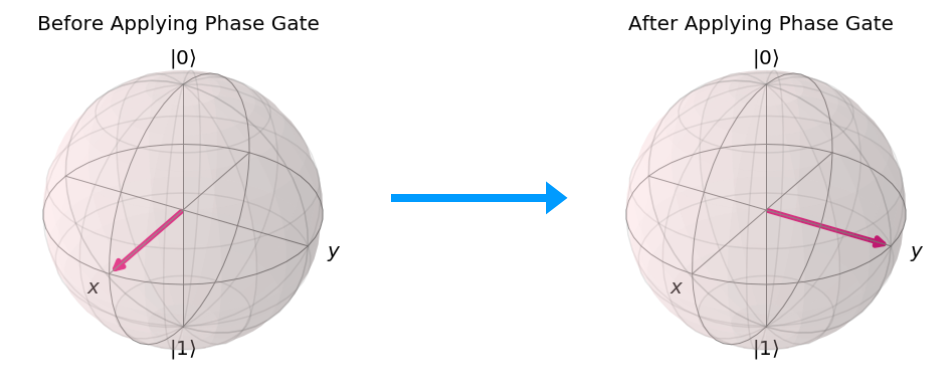
In the below figure, the Phase Gate is applied on a Qubit in the state Ψ = |->
with the parameter π/2
and the resulting state of the Qubit is Ψ = |-i>.
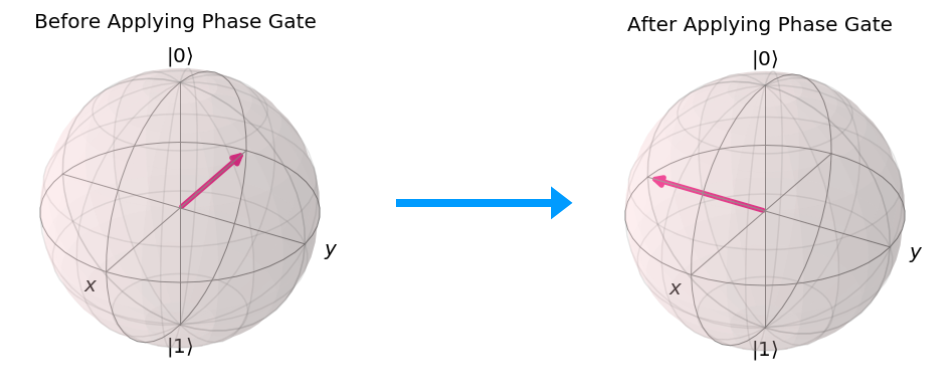
Note– Since Phase Gate actually performs rotation about the Z-axis, it will have no effect on a vector that lies on the Z-axis.
Phase Gate: Matrix
The Phase Gate in Quantum Computing is represented by the matrix P
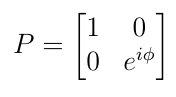
In the matrix, i represents square-root of -1 and ɸ represents the angle phase difference which is required to be applied
The resulting state of a Qubit after the application of Phase Gate can also be calculated by multiplying the Matrix for Phase Gate with the vector representing the state of the Qubit.
Example
In this example, we will apply the P gate to a Qubit, and calculate the resulting state by Multiplying it with the Matrix for P Gate.
Let the state of the Qubit be Ψ = |+> = 1/sqrt(2)|0> + 1/sqrt(2) |1>
and ɸ = π/2
.
After Applying the P Gate, the resulting state of the Qubit can be calculated by-
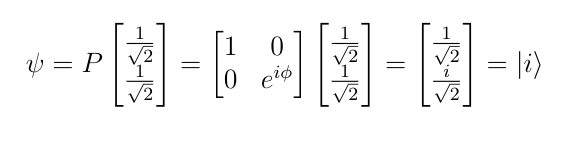
Note– The value of ei(π/2)
is i
.
Similarly as before, the probability of getting |0>
and |1>
are the same whereas there is a change in the phase of the Qubit.
Inverse of Phase Gate
The inverse of a P Gate with parameter ɸ is a P Gate with parameter -ɸ. A P Gate with parameter ɸ performs a rotation around the Z-axis of Bloch Sphere by ɸ radians in counter-clockwise direction and a P Gate with parameter -ɸ performs a rotation of ɸ radians in clockwise direction. Since applying P Gate with parameter -
on a Qubit after applying P Gate with parameter ɸ
results in the same state of the Qubit. Therefore, the inverse of a P Gate with parameter ɸ
is a P Gate with parameter ɸ
-
.ɸ
Example
For a Qubit in state
, the application of P Gate will change the state of the Qubit to Ψ = |+> = 1/sqrt(2)|0> + 1/sqrt(2) |1>
and ɸ = π/2
Ψ = 1/sqrt(2) |0> + i/sqrt(2) |1>
.
After application of the P Gate again to the same Qubit but with
, the resulting state of the Qubit can be calculated by-ɸ = -π/2
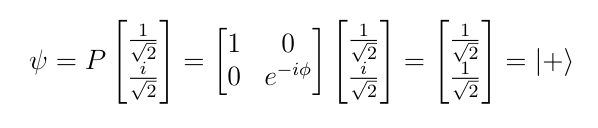
The resulting state of the Qubit will be Ψ = 1/sqrt(2) |0> + 1/sqrt(2) |1>
.
Note– The value of e-i(π/2)
is -i
.
Phase Gate in Qiskit
The Phase Gate in Qiskit can be applied to any Qubit by calling the p()
method on the Quantum Circuit(an instance of QuantumCircuit
class). Since the p()
method is parameterized, we need to pass it the phase(in radians) which needs to be applied to the Qubit on which the gate is applied.
Example
In this example, we will be applying Phase Gate with parameter π/2
on the first Qubit in the Quantum Circuit, which will contain 2 Qubits.
# Creating a Quantum Register with 1 Qubit
qr = qiskit.QuantumRegister(2)
# Creating a Quantum Circuit
circuit = QuantumCircuit(qr)
# Applying P Gate on the first Qubit
circuit.p(pi/2, 0)
# Drawing the Quantum Circuit
circuit.draw()
This will result in the following Quantum Circuit being drawn-
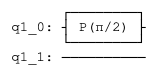
Notice that the P Gate is applied to the first Qubit. Also notice, that Qubits follow a 0 based indexing.