The RY Gate is an important gates in Quantum Computing. Qiskit provides a method for applying RY Gate on a Qubit. In this chapter of the Qiskit Tutorial, you will learn about RY Gate and how to apply RY Gate on a Qubit in Qiskit.
RY Gate
The RY Gate acts on a single Qubit. The RY Gate performs the rotation about the Y-axis of the Bloch Sphere by a given amount. It is a parameterized Gate with the parameter being the amount of rotation to be performed around the Y-axis. For example, when a RY Gate with a parameter π/4 is applied on a Qubit, the rotation about the Y-axis will be π/4 radians.
Note– The Y Gate is a special case of RY Gate when the rotation about the Y-axis of the Bloch Sphere is π radians.
RY Gate: Bloch Sphere
The RY Gate performs a rotation about the Y-axis on the Bloch Sphere by the given amount.
Example
In this example, we will look at how the application of RY gate on the Qubit affects its representation on the Bloch Sphere.
In the below figure, the RY Gate with parameter π/2 is applied on a Qubit in the state Ψ = |0>
, and the resulting state of the Qubit is
.Ψ = 1/sqrt(2) |0> + 1/sqrt(2) |1>
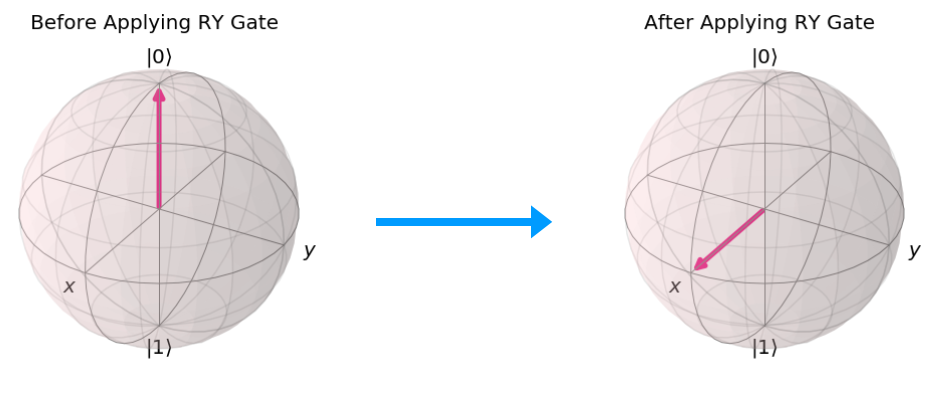
Note– Since RY Gate performs rotation about the Y-axis, it will have no effect on a vector that lies on the Y-axis.
RY Gate: Pauli Matrix
The RY Gate in Quantum Computing is represented by the matrix RY
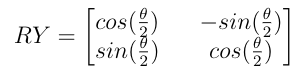
where θ is the angle of rotation about the Y axis in the counter-clockwise direction.
The resulting state of a Qubit after the application of RY Gate can also be calculated by multiplying the Matrix for RY Gate with the vector representing the state of the Qubit.
Example
In this example, we will apply the RY gate to a Qubit, and calculate the resulting state by multiplying it with the Pauli Matrix for RY Gate. The value of parameter θ will be π/2.
Let the state of the Qubit be Ψ = |0>
After Applying the RY Gate with θ = π/2, the resulting state of the Qubit can be calculated by-

Inverse of RY Gate
The inverse of RY Gate with parameter θ is RY Gate with parameter -θ . The RY Gate with parameter θ will perform a rotation around the Y-axis on the Bloch Sphere by θ radians in the counter-clockwise direction. The RY Gate with parameter -θ will perform a rotation around the Y-axis on the Bloch Sphere by θ radians in the clockwise direction.
RY Gate in Qiskit
The RY Gate in Qiskit can be applied to any Qubit by calling the ry()
method on the Quantum Circuit(an instance of QuantumCircuit
class) and passing it the amount of rotation(in radians), and an integer for the Qubit on which RY Gate is to be applied.
Example
In this example, we will be applying RY Gate on the first Qubit in the Quantum Circuit, which will contain 2 Qubits. The parameter θ is passed to the ry()
method along with the index of the Qubit on which the RY Gate is to applied. In this example, the value of θ will be π/2.
Note– The parameter θ is the angle of rotation in radians in the counter-clockwise direction.
import numpy as np
# Creating a Quantum Circuit with 2 Qubits
circuit = QuantumCircuit(2)
# Applying RY Gate on the first Qubit with parameter pi/2
circuit.ry(np.pi/2, 0)
# Drawing the Quantum Circuit
circuit.draw()
This will result in the following Quantum Circuit being drawn-
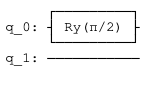