In this chapter, you will learn about Pillow ImageDraw module. Pillow allows you to draw on an image using the ImageDraw module. This module provides simple 2D graphics for Image
objects and can be used to create new images, annotate images with text or add graphics to existing images.
You need to import the ImageDraw
module to make use of these classes.
from PIL import ImageDraw
Draw class
The Draw
class is used to create an object that can be used to draw on the image. The object returned will be an instance of the class ImageDraw.ImageDraw
. Hence, all the modifications are made in place.
# creating an object of Draw class using the original image
draw = ImageDraw.Draw(img)
print(type(draw))
<class 'PIL.ImageDraw.ImageDraw'>
line()
ImageDraw.ImageDraw.line()
is used to draw a line between 2 points on the image. line()
accepts 3 arguments- xy, fill, and width.
To draw a line between points x1, y1
and x2, y2
, the coordinates can be passed as a sequence of two tuples like [(x1, y1), (x2, y2)]
or as a single sequence like [x1, y1, x2, y2]
. The xy parameter accepts the coordinates, but it is usually passed as a positional argument. The fill and width parameter passed to line()
determine the color and width of the line respectively. The default values of fill and width are ‘white’ and 1(pixel) respectively.
Syntax:
ImageDraw_obj.line(xy, fill, width)
Example
# Draws a red line between 0,0 and 400, 400 having a width of 10 pixels
draw.line([(0, 0), (400, 400)], fill='red', width=10)
# Draws a green line between 500,500 and 900, 900 having a width of 20 pixels
draw.line([500, 500, 900, 900], fill='green', width=20)
img.show()
The output of this will be-
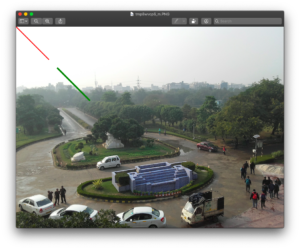
point()
ImageDraw.ImageDraw.point()
is used to ‘draw’ or ‘mark’ a point on the image. A point occupies a single pixel in the image. point()
accepts 2 arguments- xy and fill.
To draw a point x, y on the image, the coordinates can be passed as a sequence like [x, y]
or as a sequence containing a single tuple like [(x, y)]
. The xy and fill parameter passed to point()
determines the coordinates and the color of the point. The default value of fill is ‘white’.
Syntax:
ImageDraw_obj.point(xy, fill)
Example
# Draws a point at 450, 450 having blue color
draw.point([(0, 0), (400, 400)], fill='blue')
img.show()
The output of this will be-
Output Image Output Image(Zoomed)
Note– Notice that all the changes are being made to the original image and keep on getting added.
rectangle()
ImageDraw.ImageDraw.rectangle()
is used to draw a rectangle on the image. rectangle()
accepts 3 arguments- xy, fill, and outline.
To draw a rectangle defined by the bounding box points x1, y1
(top-left corner) and x2, y2
(bottom-right corner), the coordinates can be passed as a sequence of two tuples like [(x1, y1), (x2, y2)]
or as a single sequence like [x1, y1, x2, y2]
. The xy parameter accepts the coordinates, the fill and outline parameter determine the color and outline of the rectangle respectively. The default value of both is ‘white’.
Syntax:
ImageDraw_obj.rectangle(xy, fill, outline)
Example
# Draws a yellow rectangle with black outline defined by bounding box points 600, 200 and 900, 400
draw.rectangle([(600, 200), (900, 400)], fill='yellow', outline='black')
img.show()
The output of this will be-
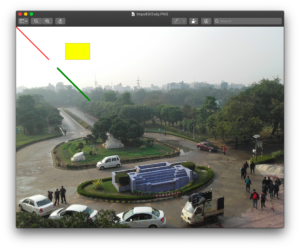
ellipse()
ImageDraw.ImageDraw.ellipse()
is used to draw an ellipse on the image. It can also be used to draw a circle since a circle is just a special case of an ellipse- when the major and minor axes are the same. ellipse()
accepts 3 arguments- xy, fill, and outline.
To draw an ellipse defined by the bounding box points x1, y1
(top-left corner) and x2, y2
(bottom-right corner), the coordinates can be passed as a sequence of two tuples like [(x1, y1), (x2, y2)]
or as a single sequence like [x1, y1, x2, y2]
. The xy parameter accepts the coordinates, the fill and outline parameter determine the color and outline of the ellipse respectively. The default value of both is ‘white’.
Syntax:
ImageDraw_obj.ellipse(xy, fill, outline)
Example
# Draws a gray ellipse with no outline defined by bounding box points 1500, 300 and 1800, 500
draw.ellipse([1500, 300, 1800, 500], fill='gray')
img.show()
The output of this will be-
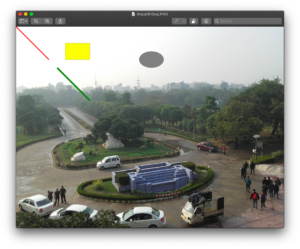
polygon()
ImageDraw.ImageDraw.polygon()
is used to draw a polygon on the image. polygon()
accepts 3 arguments- xy, fill, and outline.
To draw a polygon with points (x1, y1)
, (x2, y2)
, … , (xn, yn)
– we pass the points (x1, y1)
, (x2, y2)
, … , (xn, yn)
to polygon()
. The coordinates can be passed as a sequence of tuples like [(x1, y1), (x2, y2), ... , (xn, yn)]
or as a single sequence like [x1, y1, x2, y2, ..., xn, yn]
. The xy parameter accepts the coordinates, the fill and outline parameter determine the color and outline of the polygon respectively. The default value of both is ‘white’.
Syntax:
ImageDraw_obj.polygon(xy, fill, outline)
Example
# Draws a polygon with a red outline and white fill defined by the points passed as a sequence of tuples
draw.polygon([(2500, 400), (2900, 400), (2900,250), (2700, 300),(2500, 250)], fill='white', outline='red')
img.show()
The output of this will be-
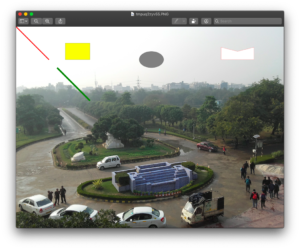
text()
ImageDraw.ImageDraw.text()
is used to ‘draw’ or ‘write’ a string on the image. The xy parameter accepts the coordinates of the top-left corner of the text, the text and fill parameters determine the content(the string which is to be drawn/ written) and color of the text respectively.
Syntax:
ImageDraw_obj.text(xy, text, fill)
Example
# Writes the given text at the given location
draw.text([(2900, 2500)], "The Photograph was taken at NSIT, Delhi", fill='red')
img.show()
The output of this will be-
Output Image Output Image(zoomed)
Note– Since the font parameter was not passed to text()
, it used default font style and font size. This can be altered by passing the required font type and font size by making use of the Pillow ImageFont
module.