In this chapter, you will be learning about Pillow ImageEnhance module. Pillow ImageEnhance module allows you to enhance the color, contrast, brightness, and sharpness of an image. These enhancements can be performed by using the classes present in the ImageEnhance
module.
You need to import the ImageEnhance
module to make use of these classes.
from PIL import ImageEnhance
_Enhance Interface
All the classes inside ImageEnhance
module implement the _Enhance
interface. The interface contains only one method, enhance()
. All the classes in the ImageEnhance
module therefore implement this method. enhance()
is callable on all objects instantiated from the classes of ImageEnhance
module. The method accepts enhancement factor as an argument and returns an image enhanced by the factor. enhance()
works in a similar fashion for all the classes inside the module.
Contrast Enhancement
The contrast of an image can be altered using the Contrast
class of the ImageEnhance
module.
The enhance()
method is passed the enhancement factor as an argument. It returns a copy of the image with the required contrast enhancement. An enhancement Factor of 0 will give a gray image with RGB value of (136, 136, 136)
. Whereas an enhancement factor of 1 will return a copy of the original image.
Example
# creating an object of Contrast class using the original image
contrast = ImageEnhance.Contrast(img)
# calling enhance() method with enhancement factor of 0.3
contrast_enhanced_img = contrast.enhance(0.3)
print(type(contrast))
# Outputs- <class 'PIL.ImageEnhance.Contrast'>
print(type(contrast_enhanced_img))
# Outputs- <class 'PIL.Image.Image'>
contrast_enhanced_img.show()
The output of this will be-
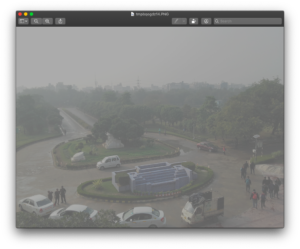
The image below compares variations of the same image with contrast enhancement factors between 0.2 to 2 with an interval of 0.2.
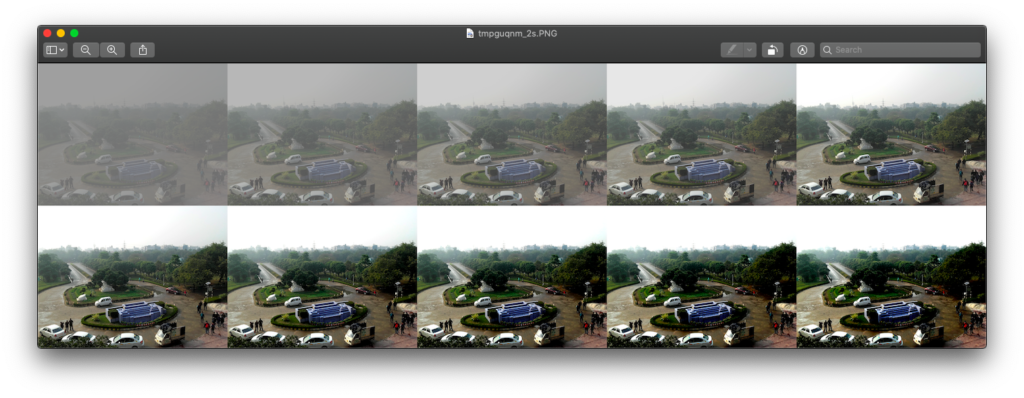
Color Enhancement
The color balance of an image can be altered using the Color
class of the ImageEnhance
module.
The enhance()
method is passed the enhancement factor as an argument. It returns a copy of the image with the required color enhancement. An enhancement Factor of 0 will give a black and white image. Whereas an enhancement factor of 1 will return a copy of the original image.
Example
# creating an object of Color class using the original image
color = ImageEnhance.Color(img)
# calling enhance() method with enhancement factor of 0.3
color_enhanced_img = color.enhance(0.3)
print(type(color))
# Outputs- <class 'PIL.ImageEnhance.Color'>
print(type(color_enhanced_img))
# Outputs- <class 'PIL.Image.Image'>
color_enhanced_img.show()
The output of this will be-
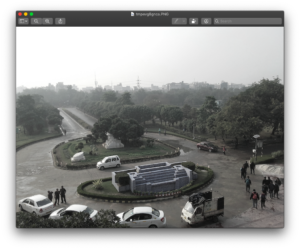
The image below compares variations of the same image with color enhancement factors between 0.2 to 2 with an interval of 0.2.
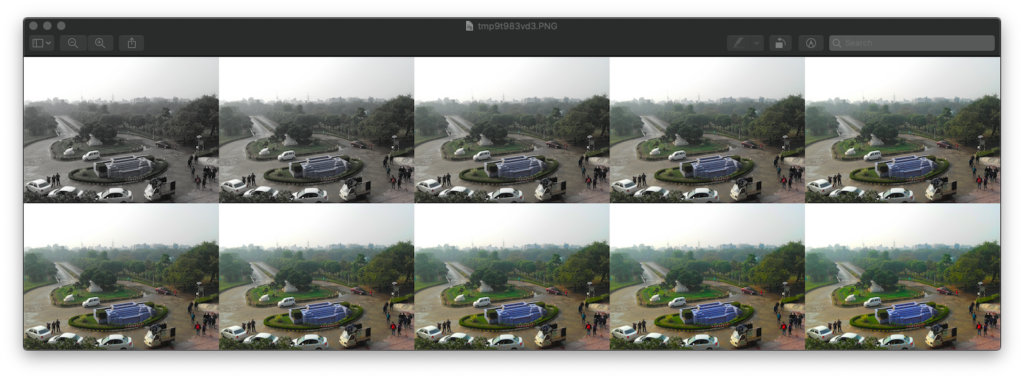
Brightness Enhancement
The brightness of an image can be altered using the Brightness
class of the ImageEnhance
module.
The enhance()
method is passed the enhancement factor as an argument. It returns a copy of the image with the required brightness enhancement. An enhancement Factor of 0 will give a black image. Whereas an enhancement factor of 1 will return a copy of the original image.
Example
# creating an object of Brightness class using the original image
brightness = ImageEnhance.Brightness(img)
# calling enhance() method with enhancement factor of 0.5
brightness_enhanced_img = brightness.enhance(0.5)
print(type(brightness))
# Outputs- <class 'PIL.ImageEnhance.Brightness'>
print(type(brightness_enhanced_img))
# Outputs- <class 'PIL.Image.Image'>
brightness_enhanced_img.show()
The output of this will be-
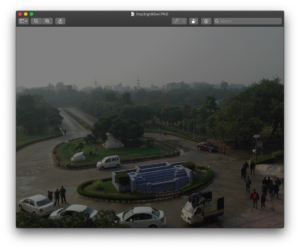
The image below compares variations of the same image with brightness enhancement factors between 0.2 to 2 with an interval of 0.2.
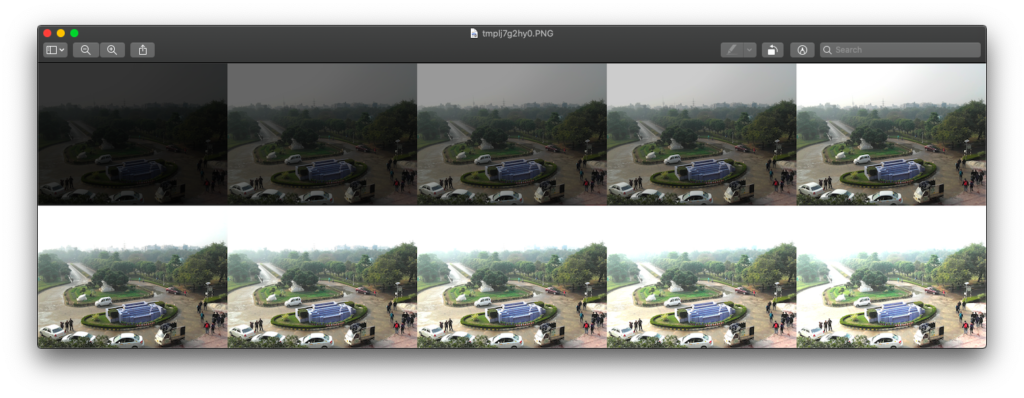
Sharpness Enhancement
The sharpness of an image can be altered using the Brightness
class of the ImageEnhance
module.
The enhance()
method is passed the enhancement factor as an argument. It returns a copy of the image with the required sharpness enhancement. An enhancement Factor of 0 will give a very blurred image. Whereas an enhancement factor of 1 will return a copy of the original image.
Example
# creating an object of Brightness class using the original image
sharpness = ImageEnhance.Sharpness(img)
# calling enhance() method with enhancement factor of 0
sharpness_enhanced_img = sharpness.enhance(0)
print(type(sharpness))
# Outputs- <class 'PIL.ImageEnhance.Sharpness'>
print(type(brightness_enhanced_img))
# Outputs- <class 'PIL.Image.Image'>
sharpness_enhanced_img.show()
The output of this will be-
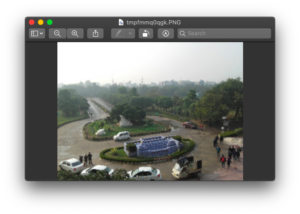
The image below compares variations of the same image with brightness enhancement factors between 0.2 to 2 with an interval of 0.2.
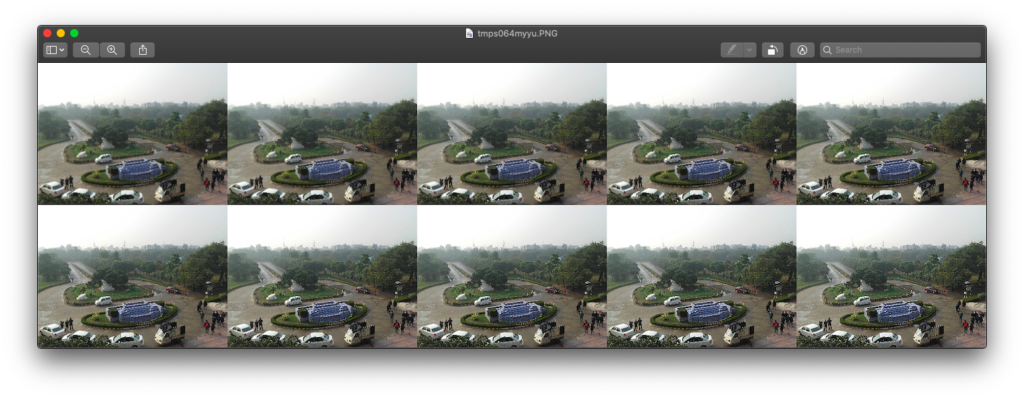