In this chapter, you will learn about Pillow ImageOps module. Pillow ImageOps module contains some ready to use image processing operations. Since this module is experimental, most of the functions provided in this module will only on images of RGB and L mode. This module can be used to add some fancy effects to the images.
You need to import the ImageOps
module to make use of these classes.
from PIL import ImageOps
flip()
ImageOps.flip()
flips the image vertically(about the horizontal axis) and returns it.
Syntax:
ImageOps.flip(image)
Example
# Flip the image img vertically and save it as flipped_img
flipped_img = ImageOps.flip(img)
flipped_img.show()
The output of this will be-
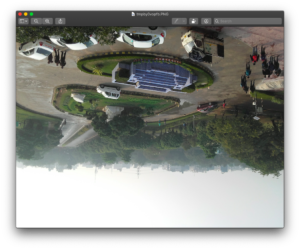
mirror()
ImageOps.mirror()
flips the image horizontally(about the vertical axis) and returns it.
Syntax:
ImageOps.mirror(image)
Example
# Flip the image img horizontally and save it as mirrored_img
mirrored_img = ImageOps.mirror(img)
mirrored_img.show()
The output of this will be-
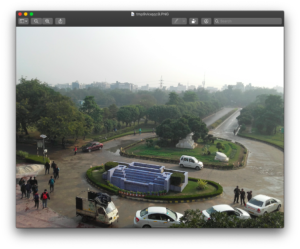
grayscale()
ImageOps.grayscale()
returns the grayscale of an image.
Syntax:
ImageOps.grayscale(image)
Example
# Convert the image to a grayscale and save it as grayscale_img
grayscale_img = ImageOps.grayscale(img)
grayscale_img.show()
The output of this will be-
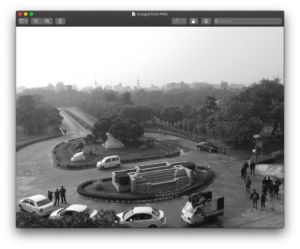
invert()
ImageOps.invert()
returns the inverted or negated of an image.
Syntax:
ImageOps.invert(image)
Example
# Convert the image to an inverted/negated image and save it as inverted_img
inverted_img = ImageOps.invert(img)
inverted_img.show()
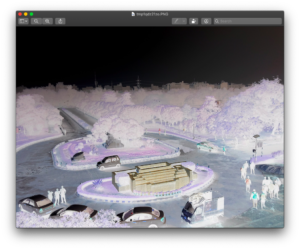
posterize()
ImageOps.posterize()
returns an image in which the number of bits is reduced for each channel.
This means that the total number of colors that can be present in the image is reduced. The number of bits that need to be present for each channel in the output image is passed to the function along with the image. The number of bits can be any number between 1-8(both inclusive).
Syntax:
ImageOps.invert(image, no_of_bits_per_channel)
Example:
# Convert the image to a posterized image and save it as posterized_img
posterized_img=ImageOps.posterize(img, 3)
# Each channel is represented by 3 bits instead of 8 in posterized_img
posterized_img.show()
The output of this will be-
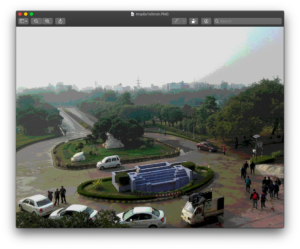
Note– Notice how the number of colors in the image has reduced as the number of bits representing a pixel of the channel is reduced to just 3 from 8. However, the image is still a color image.
The image below compares images with different numbers of bits being used for a channel. The image at the top-left uses 8 bits per channel, therefore, it is the same as the original image. Whereas the image in the bottom-right uses 1 bit per channel.
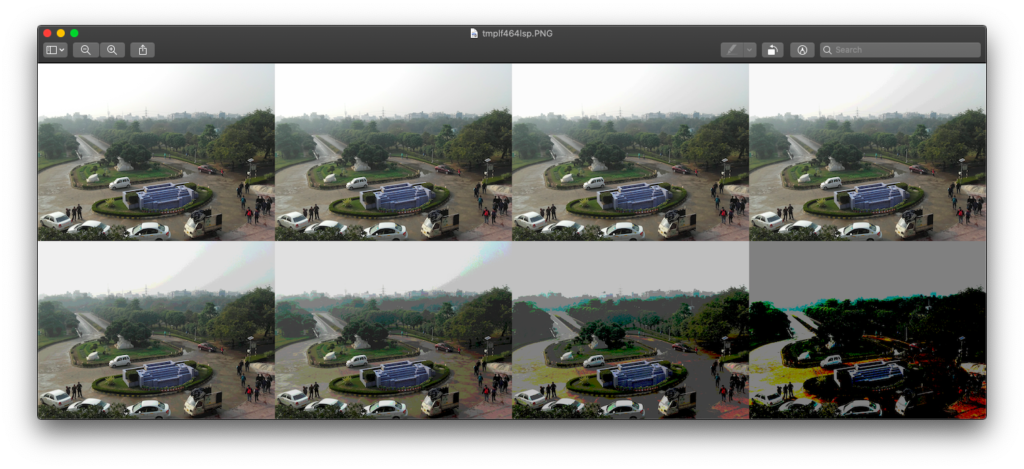
solarize()
ImageOps.solarize()
returns an image in which all the pixels values above a threshold are inverted. The threshold has a default value of 128
but can be passed as an argument along with the image.
Syntax:
ImageOps.solarize(image, threshold)
Example:
# Convert the image to a solarized image and save it as solarized_img
solarized_img=ImageOps.solarize(img, 3)
solarized_img.show()
The output of this will be-
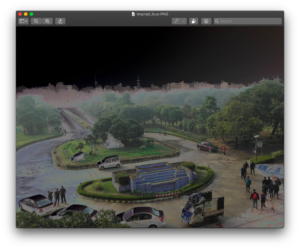